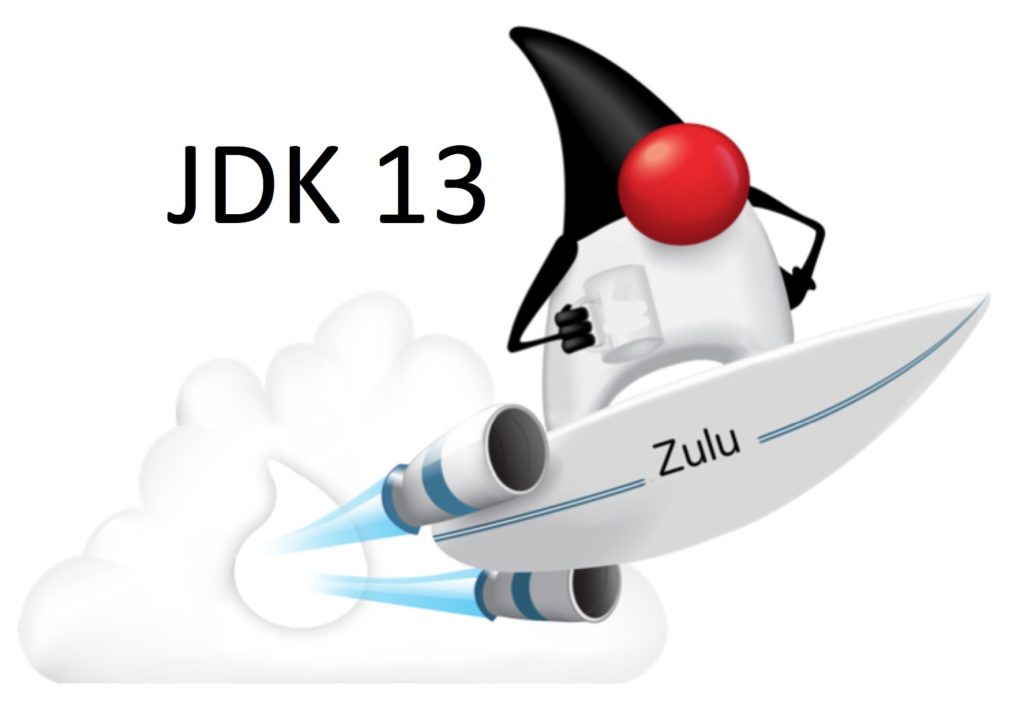
java 13 release is just around the corner, and it’s better to look into what are the new features that will be released along with it.
- Text Blocks (Preview)
- Switch Expressions (Preview)
- Re-implement the Legacy Socket API
- Dynamic CDS Archives
- ZGC: Uncommit Unused Memory
Its planned to release 5 JEP in JDK 13. As you can see above, two features are listed as preview features. That means even though the feature will be released with JDK 13, it’s not guaranteed that those features will be remain same. These features might be changed according to the feedback they get from the developers. Read more about preview features.
Let’s look into the detail to see what each feature means
Text Blocks
A text block is a multi-line string literal where it’s not required to use escape sequences. It automatically formats the String predictably and gives the developer control over format when desired.
You might have some experience of how difficult it is to embed a code snippet like Html, SQL, JSON, XML in a String literal. It requires significant effort to make it work. Even after that, it’s quite hard to read ,debug or maintain those code snippets. With new text blocks, it’s expected to address these issues.
For example , currently this is handled by using one-dimensional String literal
String html = "<html>\n" + " <body>\n" + " <p>Hello, world</p>\n" + " </body>\n" + "</html>\n";
With Java 13, this will be handled in Using a “two-dimensional” block of text
String html = """ <html> <body> <p>Hello, world</p> </body> </html> """;
Switch Expressions
Switch Expressions were initially introduced in JDK 12 as a preview feature. After collecting some feedback, they have made one change to this feature. That is adding the yield statement.
In JDK 12 , Switch expressions were introduced to address several flaws in the existing switch statement. One is, having to write unnecessary break statements which can lead to several code implications such as difficulty in debugging and readability. Also, missing break statements can lead to accidental falls through.
The traditional way of writing a switch statement to print the number of wheels on the vehicle
switch (vechile) { case BICYCLE,SCOOTER: System.out.println("two"); break; case CAR,VAN: System.out.println("four"); break; default: System.out.println("many"); }
With Switch expressions, This can be written as
switch (vechile) { case BICYCLE,SCOOTER -> System.out.println("two"); case CAR,VAN -> System.out.println("four"); default -> System.out.println("many"); }
This also can be simplified as
System.out.println( switch (vechile) { case BICYCLE,SCOOTER -> "two" case CAR,VAN -> "four" default -> "many" } );
Yield value
If the right side of the switch expression needs a full block instead of a single expression, yield statement can be used to yield value
int j = switch (vechile) { case BICYCLE,SCOOTER -> 2; case CAR,VAN -> 4; default -> { int k = calculateNumberOfWheels(vechile); yield k; } };
Re-implement the Legacy Socket API
With JDK 13, the legacy java.net.Socket and java.net.ServerSocket APIs will be re-implemented in a modern and more straightforward way. Current java.net.Socket and java.net.ServerSocket API implementation go back to JDK 1.0, which is a mix between legacy and C code which makes it really hard to maintain and debug.
One of the main goals is to make it easier to use these APIs in the future. However, the previous implementation of these APIs which is 20 years old, will not be deleted to reduce the risk. A system property will be introduced to switch back to the early implementation.
Dynamic CDS Archives
Class Data Sharing (CDS) is a concept introduced to reduce the memory footprint of application and decrease the startup time. It pre-processes a set of classes to a shared archive.
When loading classes, JVM pre-process them in a way that it could fast the execution. For example, it parses the classes and store them in an internal structure, resolve links between them, etc.
JVM also pre-load some core classes like String, List, Objects, and some classes from the application into the memory to produce better execution times. These procedures takes time to execute, and it requires some memory to store them as well.
Having a shared archive with some pre-processed would reduce the memory footprint and increase the startup time of the application.
Before JDK 10, only system classes could be added to the shared archive. With JDK 10, it provided the capability to add application classes to the shared archive as well.
JDK 13 extends this feature to allow classes to be added into the archive dynamically. At the end of the application execution, the archive will get updated with the classes which were loaded to the memory during the execution. Also, It excludes the classes which were not loaded during the execution from the archive.
ZGC: Uncommit Unused Memory
ZGC stands for the Z garbage collector, which was introduced to create a scalable, low latency garbage collector.
With JDK 13, ZGC will be improved to reallocate the unused memory back to the operating system. Currently, ZGC does not release the memory back to the operating system, even its not been used for a long time. This is not optimal for an application where memory is a concern. JDK 13 address this issue by introducing the flag -XX:ZUncommitDelay where user can specify how frequently ZGC reallocate the unused memory.